728x90
⭐ jquery
- jQuery는 클릭 이벤트, 체인지 이벤트 등 웹 페이지 동작의 기능을 조작할 때 브라우저의 영향을 받지 않고 원하는 기능을 제작할 수 있습니다.
- 브라우저의 버전에 따라 작동하지 않는 코드를 jQuery로 변경해서 사용하면 브라우저 문제 없이 사용할 수 있습니다.
- https://jquery.com/
jQuery
What is jQuery? jQuery is a fast, small, and feature-rich JavaScript library. It makes things like HTML document traversal and manipulation, event handling, animation, and Ajax much simpler with an easy-to-use API that works across a multitude of browsers.
jquery.com
1. jquery install
- install
npm install jquery
- import
import $ from 'jquery';
2. 페이지 구조 생성하기
- jquery.css
@import url(https://fonts.googleapis.com/css?family=Roboto+Slab:300,400,700);
body, h1, h2, h3, h4, h5, h6, p, ul, ol, table, dl, dd {
margin: 0;
padding: 0;
}
h1 {
font-size: 16px;
}
a {
text-decoration: none;
color: #000;
}
ul, ol {
list-style: none;
}
img {
max-width: 100%;
vertical-align: top;
border: none;
}
body {
font-family: "Roboto Slab", serif;
font-size: 16px;
font-weight: 400;
}
#header {
position: fixed;
left: 0;
top: 0;
padding: 0 22px;
width: 316px;
height: 100%;
background-color: #f5f6f8;
box-sizing: border-box;
transition: left 0.4s;
}
#header .title_area {
position: relative;
margin-top: 20px;
width: 64px;
height: 40px;
line-height: 40px;
}
#header .title_area::after {
content: "";
display: block;
position: absolute;
top: 100%;
width: 100%;
height: 3px;
background-color: #f66a69;
}
#header .title_area h2 {
font-size: 15px;
font-weight: 700;
}
#menu {
margin-top: 34px;
}
#menu > ul > li {
border-bottom: 1px solid #e1e1e1;
}
#menu > ul > li > a {
display: block;
line-height: 46px;
text-transform: uppercase;
font-size: 12px;
font-weight: 400;
color: #4a4a4a;
}
#menu ul ul {
display: none;
margin-bottom: 16px;
}
#menu > ul > li.active > a {
color: #f66a69;
}
#menu > ul > li.active ul {
display: block;
}
#menu ul ul a {
display: block;
padding-left: 12px;
line-height: 36px;
text-transform: uppercase;
font-size: 12px;
color: #bfbfbf;
}
.right_area {
margin-left: 316px;
width: auto;
height: 800px;
transition: margin-left 0.4s;
}
.right_area .tab {
display: none;
margin: 12px 0 0 12px;
width: 60px;
height: 42px;
text-indent: -9999px;
background: url(/public/assets/images/ico/btn_tab.jpg) no-repeat;
}
@media only screen and (max-width: 736px) {
#header {
left: -316px;
}
#header.open {
left: 0;
}
.right_area .tab {
display: block;
}
.right_area {
margin-left: 0;
}
.right_area.open {
margin-left: 316px;
}
}
- App.js
import React, { Component } from 'react';
import Header from './js2/step4/Header';
import Content from './js2/step4/Content';
import './css/jquery.css';
class App extends Component {
render(){
return(
<div className="wrap">
<Header />
<Content />
</div>
);
}
}
export default App;
- Header.js
import React, { Component } from 'react';
import $ from 'jquery';
class Header extends Component {
componentDidMount(){
$(window).on("resize", function(){
if($(window).width() > 736 && $("#header").hasClass("open") === true){
$("#header").removeClass("open");
$(".right_area").removeClass("open");
}
});
$(".tab").on("click", function(e){
e.preventDefault();
if($(window).width() > 735) return;
if($("#header").hasClass("open") === true){
$("#header").removeClass("open");
$(".right_area").removeClass("open");
}
else{
$("#header").addClass("open");
$(".right_area").addClass("open");
}
});
$("#menu > ul > li").on("click", function(e){
e.preventDefault();
let idx=$(this).index();
for(let i=0; i<$("#menu > ul > li").length; i++){
if(i === idx){
$("#menu > ul > li").eq(i).addClass("active");
}
else{
$("#menu > ul > li").eq(i).removeClass("active");
}
}
});
}
render(){
return(
<header id="header">
<div className="title_area">
<h2>Menu</h2>
</div>
<nav id="menu">
<ul>
<li>
<a href="">homepage</a>
<ul className="sub">
<li><a href="">lorem dolor</a></li>
<li><a href="">ipsum adipiscing</a></li>
<li><a href="">tempus magna</a></li>
<li><a href="">feugiat veroeros</a></li>
</ul>
</li>
<li>
<a href="">generic</a>
<ul className="sub">
<li><a href="">lorem dolor</a></li>
<li><a href="">ipsum adipiscing</a></li>
<li><a href="">tempus magna</a></li>
<li><a href="">feugiat veroeros</a></li>
</ul>
</li>
<li><a href="">elements</a></li>
<li><a href="">submenu</a></li>
</ul>
</nav>
</header>
);
}
}
export default Header;
- Content.js
import React, { Component } from 'react';
class Content extends Component {
render(){
return(
<div className="right_area">
<a href="" className="tab">Tab Menu</a>
</div>
);
}
}
export default Content;
- 결과 - PC
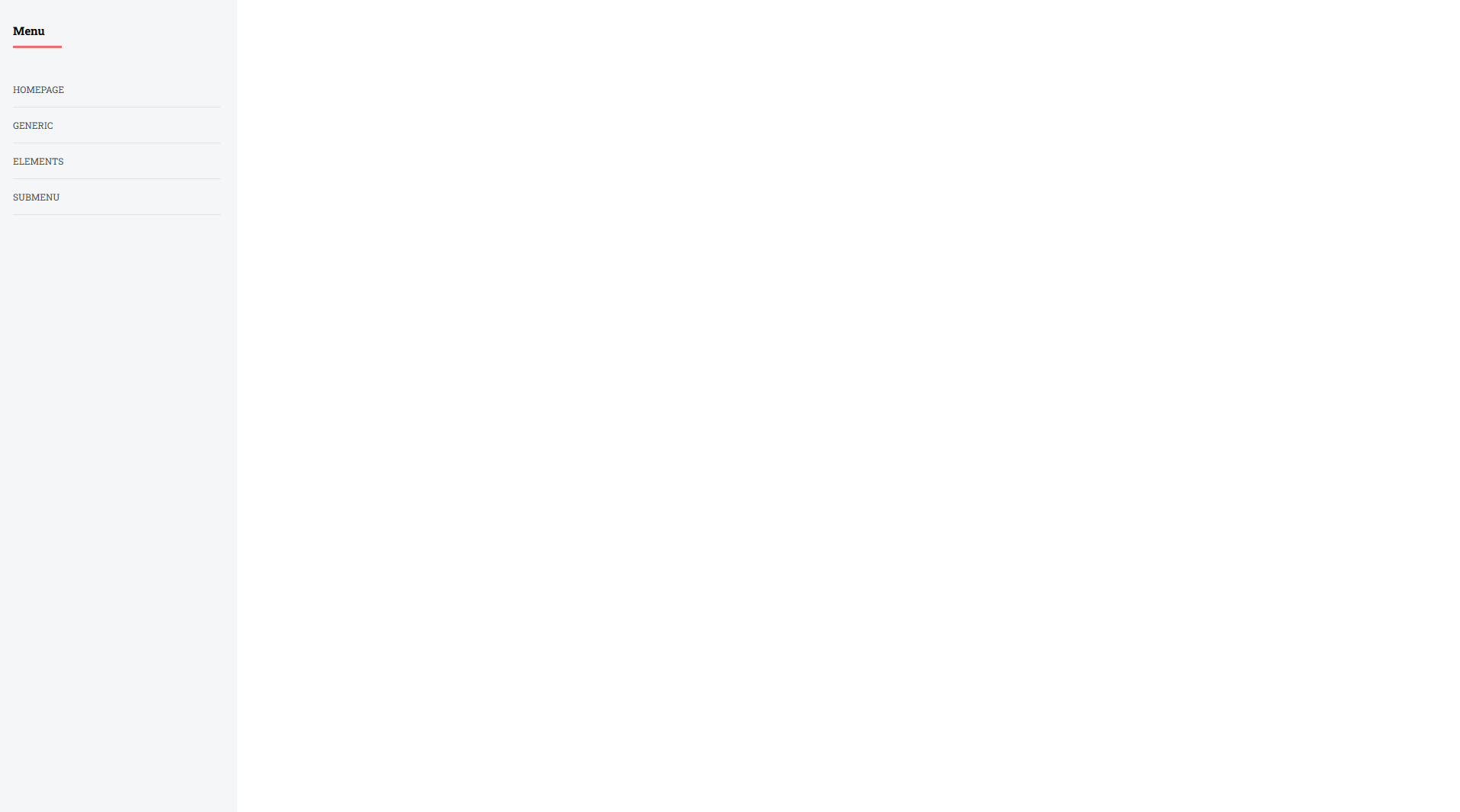
- 결과 - 모바일
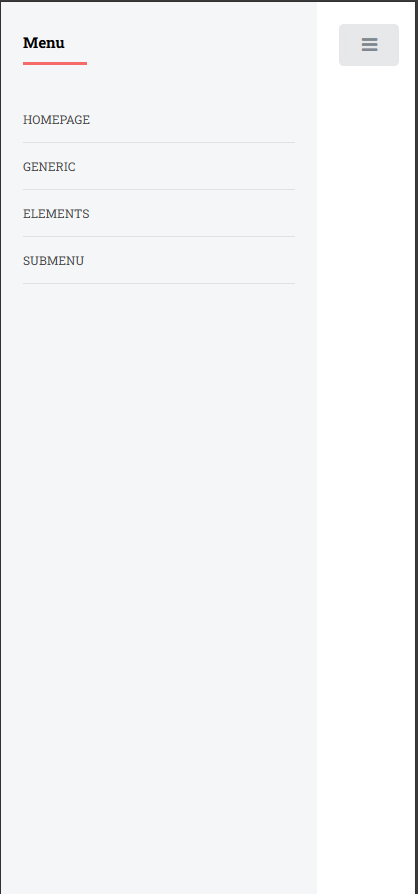
728x90
'React' 카테고리의 다른 글
React STEP 26 - gsap + react (0) | 2025.03.04 |
---|---|
React STEP 25 - 기본 구조 사용하기 (0) | 2025.03.04 |
React STEP 24 - TODO 리스트 예제 (2) | 2025.02.11 |
React STEP 23 - Create React App - 5 (1) | 2025.02.11 |
React STEP 22 - Create React App - 4 (0) | 2025.02.11 |
728x90
⭐ jquery
- jQuery는 클릭 이벤트, 체인지 이벤트 등 웹 페이지 동작의 기능을 조작할 때 브라우저의 영향을 받지 않고 원하는 기능을 제작할 수 있습니다.
- 브라우저의 버전에 따라 작동하지 않는 코드를 jQuery로 변경해서 사용하면 브라우저 문제 없이 사용할 수 있습니다.
- https://jquery.com/
jQuery
What is jQuery? jQuery is a fast, small, and feature-rich JavaScript library. It makes things like HTML document traversal and manipulation, event handling, animation, and Ajax much simpler with an easy-to-use API that works across a multitude of browsers.
jquery.com
1. jquery install
- install
npm install jquery
- import
import $ from 'jquery';
2. 페이지 구조 생성하기
- jquery.css
@import url(https://fonts.googleapis.com/css?family=Roboto+Slab:300,400,700);
body, h1, h2, h3, h4, h5, h6, p, ul, ol, table, dl, dd {
margin: 0;
padding: 0;
}
h1 {
font-size: 16px;
}
a {
text-decoration: none;
color: #000;
}
ul, ol {
list-style: none;
}
img {
max-width: 100%;
vertical-align: top;
border: none;
}
body {
font-family: "Roboto Slab", serif;
font-size: 16px;
font-weight: 400;
}
#header {
position: fixed;
left: 0;
top: 0;
padding: 0 22px;
width: 316px;
height: 100%;
background-color: #f5f6f8;
box-sizing: border-box;
transition: left 0.4s;
}
#header .title_area {
position: relative;
margin-top: 20px;
width: 64px;
height: 40px;
line-height: 40px;
}
#header .title_area::after {
content: "";
display: block;
position: absolute;
top: 100%;
width: 100%;
height: 3px;
background-color: #f66a69;
}
#header .title_area h2 {
font-size: 15px;
font-weight: 700;
}
#menu {
margin-top: 34px;
}
#menu > ul > li {
border-bottom: 1px solid #e1e1e1;
}
#menu > ul > li > a {
display: block;
line-height: 46px;
text-transform: uppercase;
font-size: 12px;
font-weight: 400;
color: #4a4a4a;
}
#menu ul ul {
display: none;
margin-bottom: 16px;
}
#menu > ul > li.active > a {
color: #f66a69;
}
#menu > ul > li.active ul {
display: block;
}
#menu ul ul a {
display: block;
padding-left: 12px;
line-height: 36px;
text-transform: uppercase;
font-size: 12px;
color: #bfbfbf;
}
.right_area {
margin-left: 316px;
width: auto;
height: 800px;
transition: margin-left 0.4s;
}
.right_area .tab {
display: none;
margin: 12px 0 0 12px;
width: 60px;
height: 42px;
text-indent: -9999px;
background: url(/public/assets/images/ico/btn_tab.jpg) no-repeat;
}
@media only screen and (max-width: 736px) {
#header {
left: -316px;
}
#header.open {
left: 0;
}
.right_area .tab {
display: block;
}
.right_area {
margin-left: 0;
}
.right_area.open {
margin-left: 316px;
}
}
- App.js
import React, { Component } from 'react';
import Header from './js2/step4/Header';
import Content from './js2/step4/Content';
import './css/jquery.css';
class App extends Component {
render(){
return(
<div className="wrap">
<Header />
<Content />
</div>
);
}
}
export default App;
- Header.js
import React, { Component } from 'react';
import $ from 'jquery';
class Header extends Component {
componentDidMount(){
$(window).on("resize", function(){
if($(window).width() > 736 && $("#header").hasClass("open") === true){
$("#header").removeClass("open");
$(".right_area").removeClass("open");
}
});
$(".tab").on("click", function(e){
e.preventDefault();
if($(window).width() > 735) return;
if($("#header").hasClass("open") === true){
$("#header").removeClass("open");
$(".right_area").removeClass("open");
}
else{
$("#header").addClass("open");
$(".right_area").addClass("open");
}
});
$("#menu > ul > li").on("click", function(e){
e.preventDefault();
let idx=$(this).index();
for(let i=0; i<$("#menu > ul > li").length; i++){
if(i === idx){
$("#menu > ul > li").eq(i).addClass("active");
}
else{
$("#menu > ul > li").eq(i).removeClass("active");
}
}
});
}
render(){
return(
<header id="header">
<div className="title_area">
<h2>Menu</h2>
</div>
<nav id="menu">
<ul>
<li>
<a href="">homepage</a>
<ul className="sub">
<li><a href="">lorem dolor</a></li>
<li><a href="">ipsum adipiscing</a></li>
<li><a href="">tempus magna</a></li>
<li><a href="">feugiat veroeros</a></li>
</ul>
</li>
<li>
<a href="">generic</a>
<ul className="sub">
<li><a href="">lorem dolor</a></li>
<li><a href="">ipsum adipiscing</a></li>
<li><a href="">tempus magna</a></li>
<li><a href="">feugiat veroeros</a></li>
</ul>
</li>
<li><a href="">elements</a></li>
<li><a href="">submenu</a></li>
</ul>
</nav>
</header>
);
}
}
export default Header;
- Content.js
import React, { Component } from 'react';
class Content extends Component {
render(){
return(
<div className="right_area">
<a href="" className="tab">Tab Menu</a>
</div>
);
}
}
export default Content;
- 결과 - PC
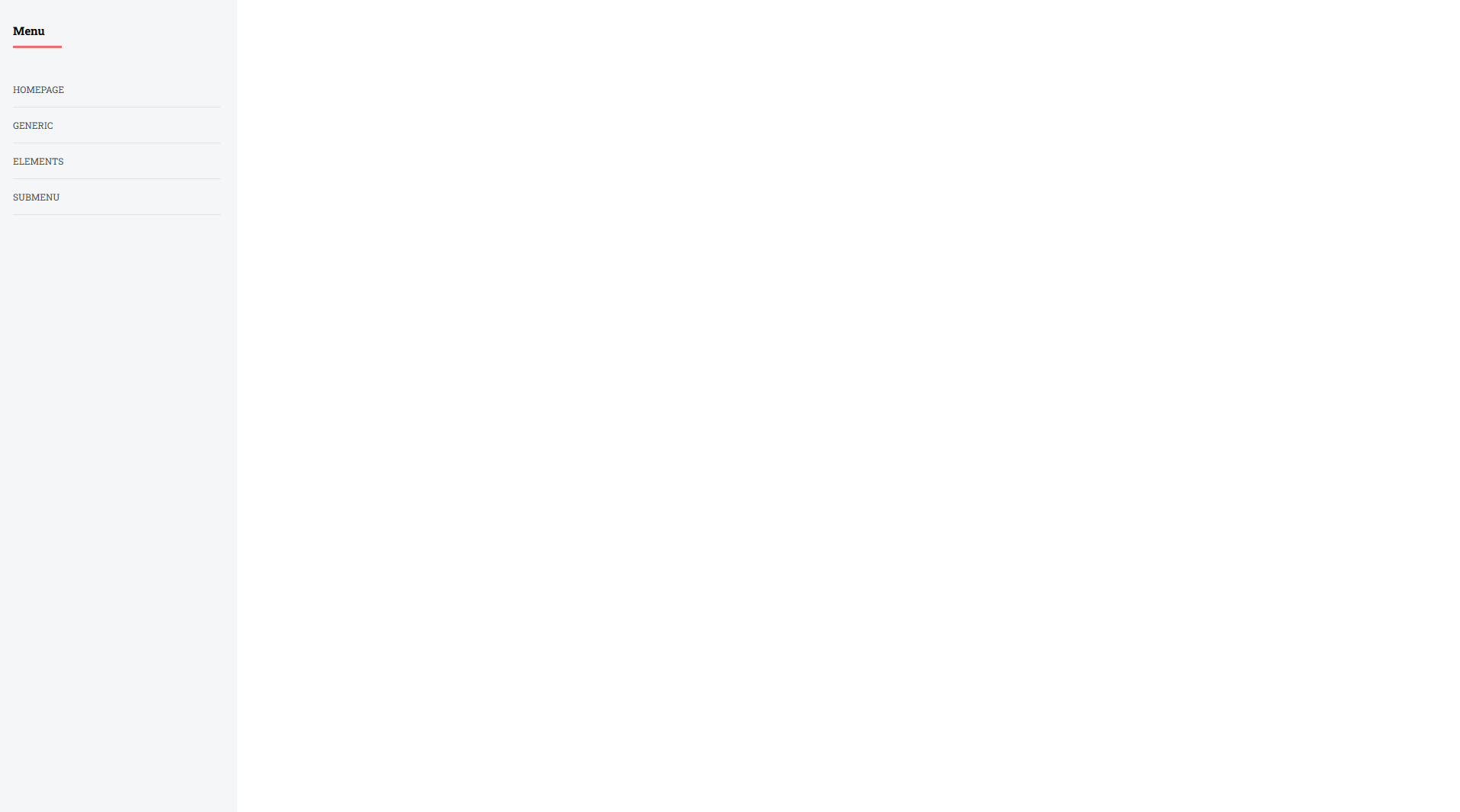
- 결과 - 모바일
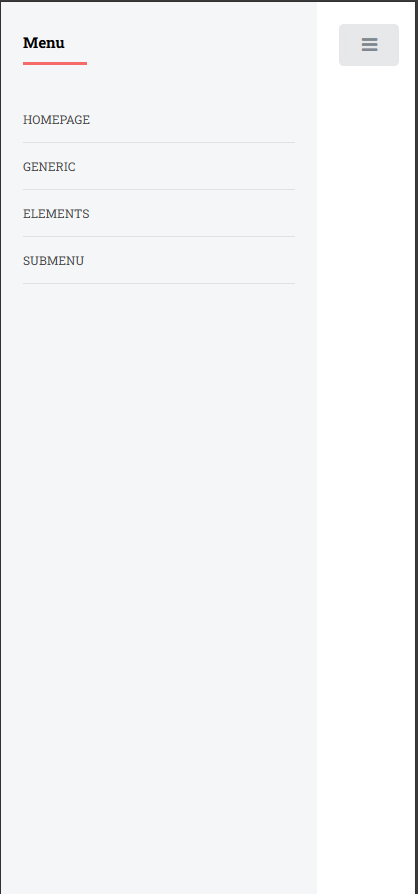
728x90
'React' 카테고리의 다른 글
React STEP 26 - gsap + react (0) | 2025.03.04 |
---|---|
React STEP 25 - 기본 구조 사용하기 (0) | 2025.03.04 |
React STEP 24 - TODO 리스트 예제 (2) | 2025.02.11 |
React STEP 23 - Create React App - 5 (1) | 2025.02.11 |
React STEP 22 - Create React App - 4 (0) | 2025.02.11 |