★ JSP 실행 과정
- 1. 경로 확인
System.out.println(request.getRealPath("."));
- 경로 결과
C:\class\code\server\.metadata\.plugins\org.eclipse.wst.server.core\tmp0\wtpwebapps\JSPTest
- 실행 구조
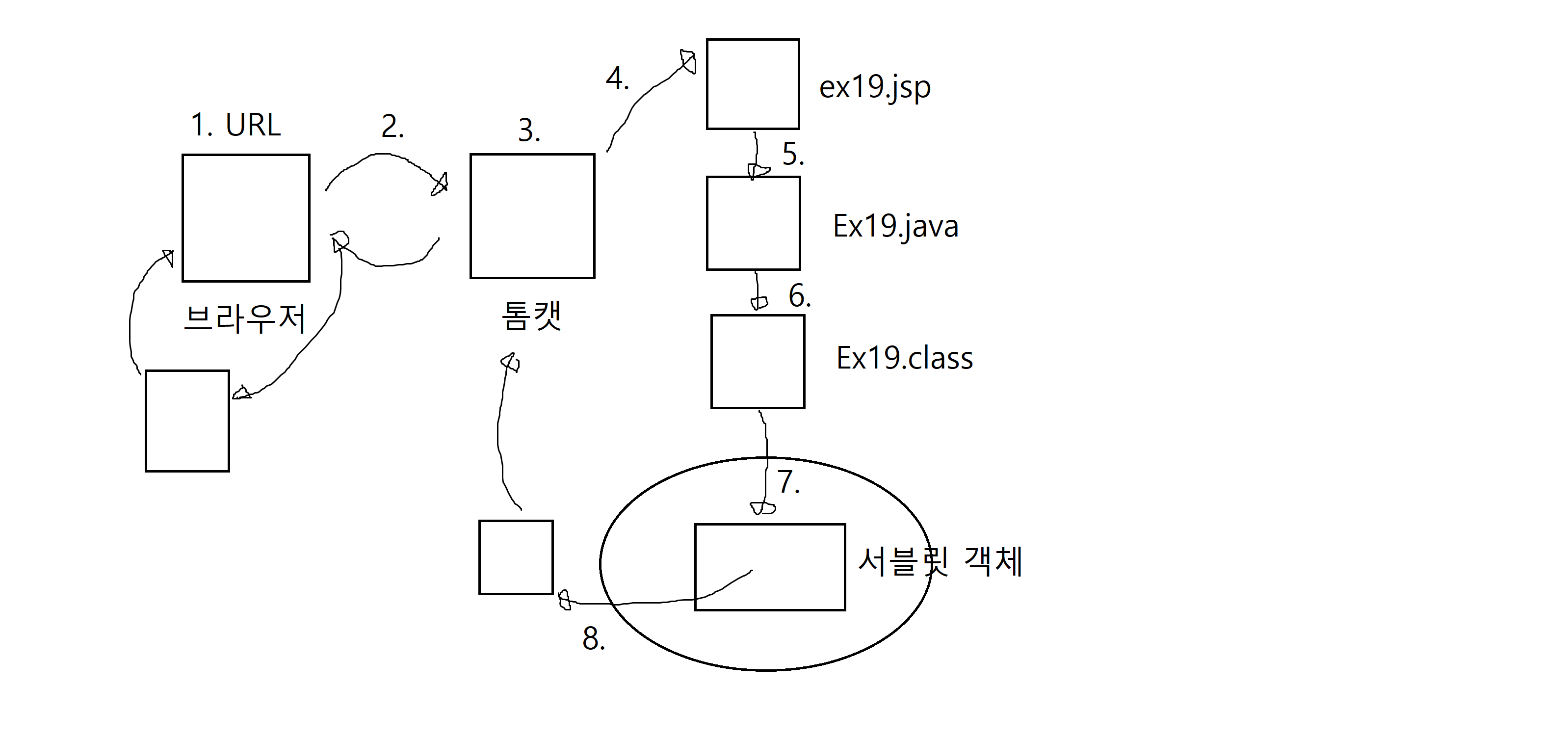
★ 쿠키, Cookie
- 개인 정보를 저장하는 저장소
- 브라우저가 관리하는 저장소
- 방문자가 사이트를 접속 > 사이트에 접속한 브라우저(개개인)의 정보를 관리하기 위해서 쿠키에 정보를 입출력
- JSP(Servlet), JavaScript 등 > 쿠키에 접근해서 조작 가능
- "지속적으로 개개인의 정보 관리"
★쿠키의 종류
- 1. 메모리 쿠키
- 브라우저 실행중에만 유지
- 브라우저가 종료되면 쿠키도 삭제
- 메모리에만 상주
- 2. 하드 쿠키
- 브라우저가 종료되도 유지
- 하드 디스크에 저장
■ get, set Cookie
JavaScript Cookies
W3Schools offers free online tutorials, references and exercises in all the major languages of the web. Covering popular subjects like HTML, CSS, JavaScript, Python, SQL, Java, and many, many more.
www.w3schools.com
- 코드
function setCookie(cname, cvalue, exdays) {
const d = new Date();
d.setTime(d.getTime() + (exdays * 24 * 60 * 60 * 1000));
let expires = "expires="+d.toUTCString();
document.cookie = cname + "=" + cvalue + ";" + expires + ";path=/";
}
function getCookie(cname) {
let name = cname + "=";
let ca = document.cookie.split(';');
for(let i = 0; i < ca.length; i++) {
let c = ca[i];
while (c.charAt(0) == ' ') {
c = c.substring(1);
}
if (c.indexOf(name) == 0) {
return c.substring(name.length, c.length);
}
}
return "";
}
■ Cookie를 이용한, 이미지 배경 변경 알고리즘
- ex20_cookie.one.jsp
- ex20_cookie.two.jsp
- ex20_cookie.three.jsp
▶ ex20_cookie.one.jsp 주요 소스코드
<body>
<h1>쿠키, Cookie</h1>
<h2>배경 화면 선택(테마)</h2>
<table>
<tr>
<td><img src="images/rect_icon01.png"></td>
<td><input type="radio" name="background" value="rect_icon01.png" checked></td>
</tr>
<tr>
<td><img src="images/rect_icon02.png"></td>
<td><input type="radio" name="background" value="rect_icon02.png"></td>
</tr>
<tr>
<td><img src="images/rect_icon03.png"></td>
<td><input type="radio" name="background" value="rect_icon03.png"></td>
</tr>
</table>
<hr>
<div style="background-color : white;">
<a href="ex20_cookie.one.jsp">첫번째 페이지</a>
<a href="ex20_cookie.two.jsp">두번째 페이지</a>
<a href="ex20_cookie.three.jsp">세번째 페이지</a>
</div>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.4/jquery.min.js"></script>
<script src="js/cookie.js"></script>
<script>
// document.cookie = 100;
// console.log(documnet.cookie);
// key=value&key=value..
setCookie('name', '홍길동');
console.log(getCookie('name'));
if(getCookie('background') == '') {
//첫방문
setCookie('background', 'rect_icon01.png');
} else {
//재방문
$('body').css('background-image', `url(images/\${getCookie('background')})`);
$('input[name=background]').each((index, item) => {
if($(item).val() == getCookie('background')) {
$(item).prop('checked', true);
}else {
$(item).prop('checked', false);
}
});
}
$('input[name=background]').change(function() {
//alert($(this).val());
$('body').css('background-image'
, `url(images/\${$(this).val()})`);
//선택한 테마 > 쿠키 저장
setCookie('background', $(this).val());
});
</script>
</body>
▶ ex20_cookie.two.jsp 주요 소스코드
<body>
<h1>두번째 페이지</h1>
<hr>
<div style="background-color : white;">
<a href="ex20_cookie.one.jsp">첫번째 페이지</a>
<a href="ex20_cookie.two.jsp">두번째 페이지</a>
<a href="ex20_cookie.three.jsp">세번째 페이지</a>
</div>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.4/jquery.min.js"></script>
<script src="js/cookie.js"></script>
<script>
$('body').css('background-image', `url(images/\${getCookie('background')})`);
</script>
</body>
▶ ex20_cookie.thress.jsp 주요 소스코드
<body>
<h1>세번째 페이지</h1>
<hr>
<div style="background-color : white;">
<a href="ex20_cookie.one.jsp">첫번째 페이지</a>
<a href="ex20_cookie.two.jsp">두번째 페이지</a>
<a href="ex20_cookie.three.jsp">세번째 페이지</a>
</div>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.4/jquery.min.js"></script>
<script src="js/cookie.js"></script>
<script>
$('body').css('background-image', `url(images/\${getCookie('background')})`);
</script>
</body>
■ 실행 결과
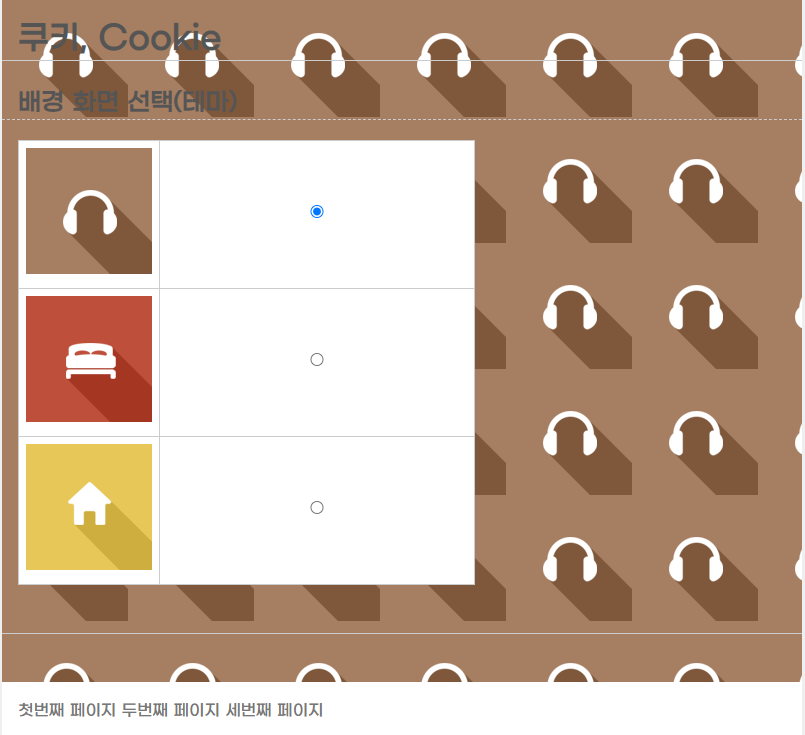
■ Cookie를 이용한, id기억하기 알고리즘
- ex21_cookie.jsp
- 주요 소스코드
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<link rel="stylesheet" href="https://me2.do/5BvBFJ57">
<style>
#txtid, #txtpw {
width : 120px;
}
table th {
width : 120px !important;
}
table td {
width : 180px !important;
}
</style>
</head>
<body>
<h1>로그인</h1>
<table class="vertical" style="width:300px;">
<tr>
<th>아이디</th>
<td><input type="text" id="txtid"></td>
</tr>
<tr>
<th>비밀번호</th>
<td><input type="password" id="txtpw"></td>
</tr>
</table>
<div>
<input type="checkbox" id="cb"> 아이디 기억하기
<input type="button" value="로그인" id="btn">
</div>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.4/jquery.min.js"></script>
<script src="js/cookie.js"></script>
<script>
$('#btn').click(function() {
//로그인
if ($('#txtid').val() == 'hong' && $('#txtpw').val() == '1234') {
location.href = 'ex20_cookie.one.jsp'
if($('#cb').prop('checked')) {
//alert('O');
setCookie('id', $('#txtid').val(), 365);
} else {
alert('X');
setCookie('id', '', -1); //어제
}
}else {
alert('아이디 or 암호가 일치하지 않습니다.');
}
});
if(getCookie('id') != '') {
//id가 있으면
$('#txtid').val(getCookie('id'));
$('#txtpw').focus();
$('#cb').prop('checked', true);
} else {
$('#txtid').focus();
};
</script>
</body>
</html>
- 실행 결과
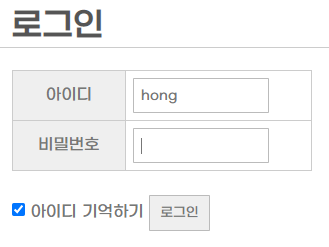
'Server' 카테고리의 다른 글
JSP STEP 9 - Web Security (0) | 2023.05.10 |
---|---|
JSP STEP 8 - DB를 연동하여, 주소록 사이트 만들기 (2) | 2023.05.10 |
JSP STEP 6 - Application (0) | 2023.05.09 |
JSP STEP 5 - Session (1) | 2023.05.09 |
JSP STEP 4 - Response (1) | 2023.05.09 |
★ JSP 실행 과정
- 1. 경로 확인
System.out.println(request.getRealPath("."));
- 경로 결과
C:\class\code\server\.metadata\.plugins\org.eclipse.wst.server.core\tmp0\wtpwebapps\JSPTest
- 실행 구조
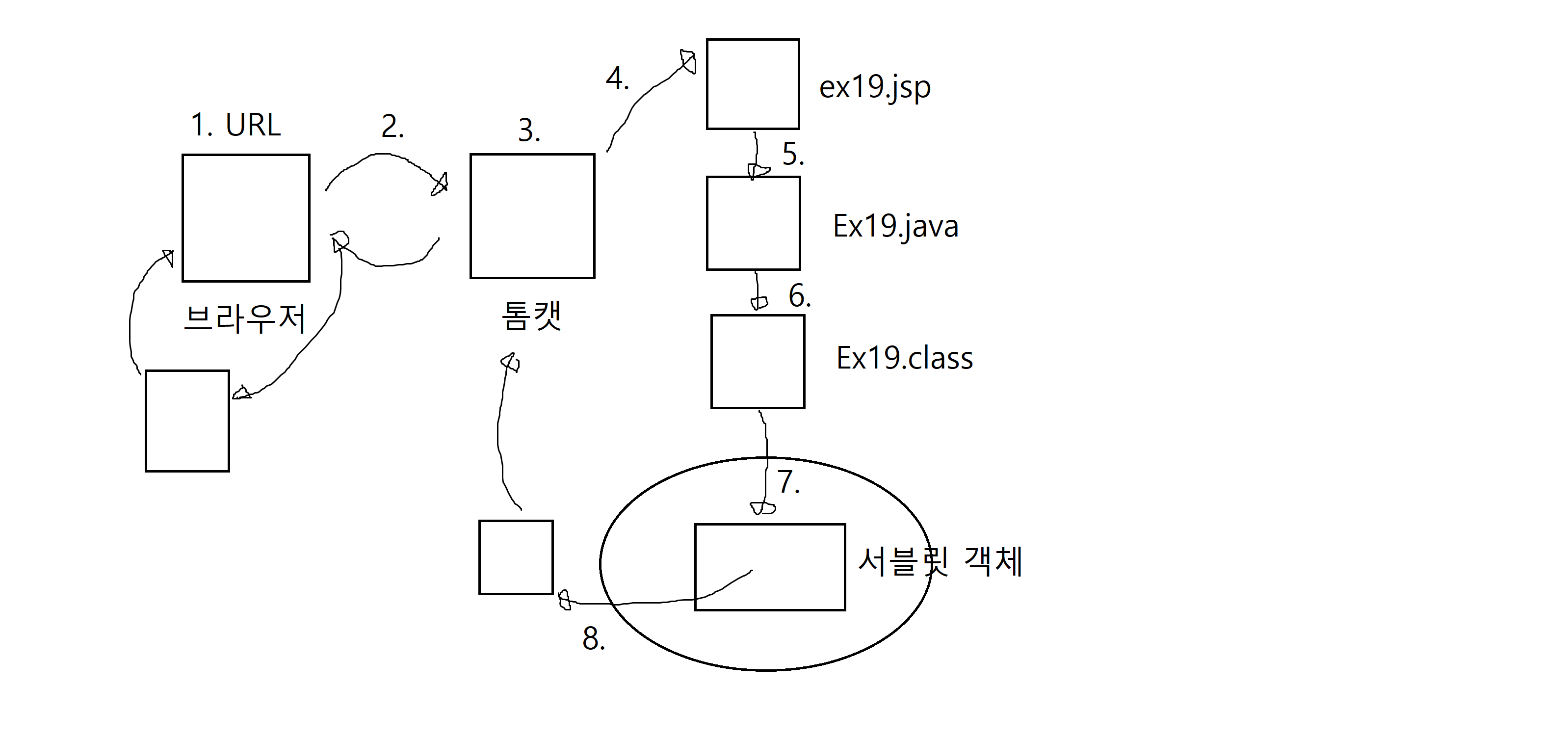
★ 쿠키, Cookie
- 개인 정보를 저장하는 저장소
- 브라우저가 관리하는 저장소
- 방문자가 사이트를 접속 > 사이트에 접속한 브라우저(개개인)의 정보를 관리하기 위해서 쿠키에 정보를 입출력
- JSP(Servlet), JavaScript 등 > 쿠키에 접근해서 조작 가능
- "지속적으로 개개인의 정보 관리"
★쿠키의 종류
- 1. 메모리 쿠키
- 브라우저 실행중에만 유지
- 브라우저가 종료되면 쿠키도 삭제
- 메모리에만 상주
- 2. 하드 쿠키
- 브라우저가 종료되도 유지
- 하드 디스크에 저장
■ get, set Cookie
JavaScript Cookies
W3Schools offers free online tutorials, references and exercises in all the major languages of the web. Covering popular subjects like HTML, CSS, JavaScript, Python, SQL, Java, and many, many more.
www.w3schools.com
- 코드
function setCookie(cname, cvalue, exdays) {
const d = new Date();
d.setTime(d.getTime() + (exdays * 24 * 60 * 60 * 1000));
let expires = "expires="+d.toUTCString();
document.cookie = cname + "=" + cvalue + ";" + expires + ";path=/";
}
function getCookie(cname) {
let name = cname + "=";
let ca = document.cookie.split(';');
for(let i = 0; i < ca.length; i++) {
let c = ca[i];
while (c.charAt(0) == ' ') {
c = c.substring(1);
}
if (c.indexOf(name) == 0) {
return c.substring(name.length, c.length);
}
}
return "";
}
■ Cookie를 이용한, 이미지 배경 변경 알고리즘
- ex20_cookie.one.jsp
- ex20_cookie.two.jsp
- ex20_cookie.three.jsp
▶ ex20_cookie.one.jsp 주요 소스코드
<body>
<h1>쿠키, Cookie</h1>
<h2>배경 화면 선택(테마)</h2>
<table>
<tr>
<td><img src="images/rect_icon01.png"></td>
<td><input type="radio" name="background" value="rect_icon01.png" checked></td>
</tr>
<tr>
<td><img src="images/rect_icon02.png"></td>
<td><input type="radio" name="background" value="rect_icon02.png"></td>
</tr>
<tr>
<td><img src="images/rect_icon03.png"></td>
<td><input type="radio" name="background" value="rect_icon03.png"></td>
</tr>
</table>
<hr>
<div style="background-color : white;">
<a href="ex20_cookie.one.jsp">첫번째 페이지</a>
<a href="ex20_cookie.two.jsp">두번째 페이지</a>
<a href="ex20_cookie.three.jsp">세번째 페이지</a>
</div>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.4/jquery.min.js"></script>
<script src="js/cookie.js"></script>
<script>
// document.cookie = 100;
// console.log(documnet.cookie);
// key=value&key=value..
setCookie('name', '홍길동');
console.log(getCookie('name'));
if(getCookie('background') == '') {
//첫방문
setCookie('background', 'rect_icon01.png');
} else {
//재방문
$('body').css('background-image', `url(images/\${getCookie('background')})`);
$('input[name=background]').each((index, item) => {
if($(item).val() == getCookie('background')) {
$(item).prop('checked', true);
}else {
$(item).prop('checked', false);
}
});
}
$('input[name=background]').change(function() {
//alert($(this).val());
$('body').css('background-image'
, `url(images/\${$(this).val()})`);
//선택한 테마 > 쿠키 저장
setCookie('background', $(this).val());
});
</script>
</body>
▶ ex20_cookie.two.jsp 주요 소스코드
<body>
<h1>두번째 페이지</h1>
<hr>
<div style="background-color : white;">
<a href="ex20_cookie.one.jsp">첫번째 페이지</a>
<a href="ex20_cookie.two.jsp">두번째 페이지</a>
<a href="ex20_cookie.three.jsp">세번째 페이지</a>
</div>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.4/jquery.min.js"></script>
<script src="js/cookie.js"></script>
<script>
$('body').css('background-image', `url(images/\${getCookie('background')})`);
</script>
</body>
▶ ex20_cookie.thress.jsp 주요 소스코드
<body>
<h1>세번째 페이지</h1>
<hr>
<div style="background-color : white;">
<a href="ex20_cookie.one.jsp">첫번째 페이지</a>
<a href="ex20_cookie.two.jsp">두번째 페이지</a>
<a href="ex20_cookie.three.jsp">세번째 페이지</a>
</div>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.4/jquery.min.js"></script>
<script src="js/cookie.js"></script>
<script>
$('body').css('background-image', `url(images/\${getCookie('background')})`);
</script>
</body>
■ 실행 결과
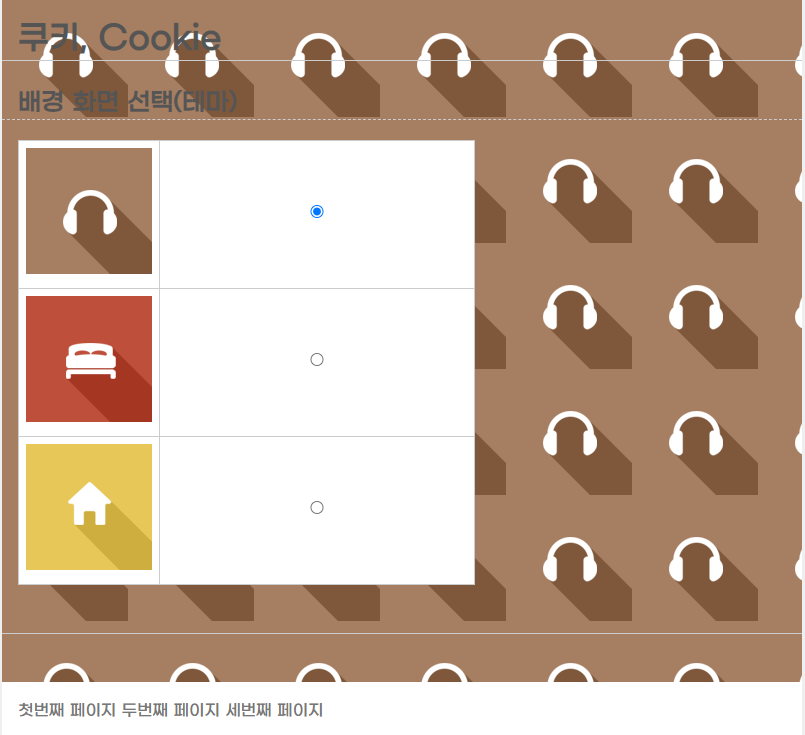
■ Cookie를 이용한, id기억하기 알고리즘
- ex21_cookie.jsp
- 주요 소스코드
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<link rel="stylesheet" href="https://me2.do/5BvBFJ57">
<style>
#txtid, #txtpw {
width : 120px;
}
table th {
width : 120px !important;
}
table td {
width : 180px !important;
}
</style>
</head>
<body>
<h1>로그인</h1>
<table class="vertical" style="width:300px;">
<tr>
<th>아이디</th>
<td><input type="text" id="txtid"></td>
</tr>
<tr>
<th>비밀번호</th>
<td><input type="password" id="txtpw"></td>
</tr>
</table>
<div>
<input type="checkbox" id="cb"> 아이디 기억하기
<input type="button" value="로그인" id="btn">
</div>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.4/jquery.min.js"></script>
<script src="js/cookie.js"></script>
<script>
$('#btn').click(function() {
//로그인
if ($('#txtid').val() == 'hong' && $('#txtpw').val() == '1234') {
location.href = 'ex20_cookie.one.jsp'
if($('#cb').prop('checked')) {
//alert('O');
setCookie('id', $('#txtid').val(), 365);
} else {
alert('X');
setCookie('id', '', -1); //어제
}
}else {
alert('아이디 or 암호가 일치하지 않습니다.');
}
});
if(getCookie('id') != '') {
//id가 있으면
$('#txtid').val(getCookie('id'));
$('#txtpw').focus();
$('#cb').prop('checked', true);
} else {
$('#txtid').focus();
};
</script>
</body>
</html>
- 실행 결과
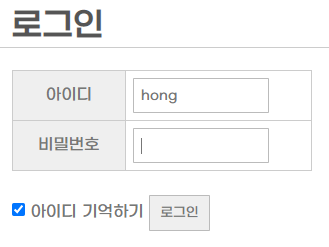
'Server' 카테고리의 다른 글
JSP STEP 9 - Web Security (0) | 2023.05.10 |
---|---|
JSP STEP 8 - DB를 연동하여, 주소록 사이트 만들기 (2) | 2023.05.10 |
JSP STEP 6 - Application (0) | 2023.05.09 |
JSP STEP 5 - Session (1) | 2023.05.09 |
JSP STEP 4 - Response (1) | 2023.05.09 |